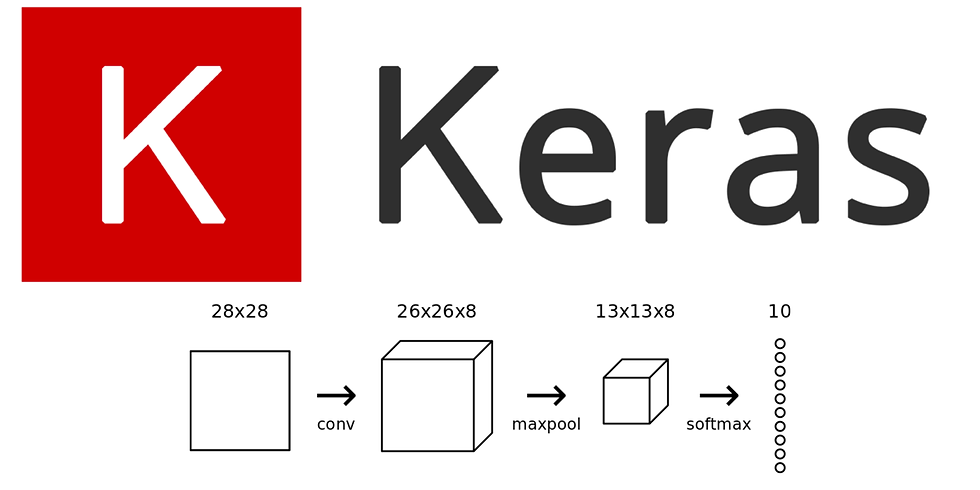
Keras is an open-source software library that provides a Python interface for ANNs (Artificial Neural Networks). It is a high-level library that runs on top of TensorFlow, CNTK, or Theano. Keras acts as an interface for the TensorFlow library. In this article, we will discuss what Keras is and its benefits, how to use it, and how it can be applied to solve various problems in the field of deep learning.
Keras was developed with a focus on enabling fast experimentation and prototyping while allowing for the support of both convolutional and recurrent networks. It has gained immense popularity in the deep learning community due to its simplicity and ease of use compared to the low-level libraries such as TensorFlow. Keras abstracts much of the complex functionality of TensorFlow and presents a more intuitive interface to the user.
One of the main benefits of Keras is its user-friendly API. The API was designed to be easy to learn and use for developers who are not familiar with deep learning. It provides a high-level, user-friendly API for defining and training deep learning models. The API has a consistent and simple interface for defining models, as well as support for model training, evaluation, and prediction.
Keras also provides several pre-trained deep learning models for image classification, object detection, and text classification that can be easily integrated into your own projects. These pre-trained models can be fine-tuned and customized for your specific use-case, which saves time and resources compared to training models from scratch.
One of the practical applications of Keras is in the field of computer vision. Keras provides a variety of pre-trained models for image classification, object detection, and image segmentation. For instance, a popular deep learning model for image classification is the VGG16 model, which has been pre-trained on the ImageNet dataset. This model can be easily loaded in Keras and fine-tuned for a specific image classification problem.
Another application of Keras is in the field of natural language processing (NLP). NLP is a field of study that focuses on the interactions between computers and humans using natural language. Keras provides several pre-trained models for text classification, sentiment analysis, and text generation. These models can be easily fine-tuned and customized for your specific use-case.
Here is an example code for building a simple multi-layer perceptron (MLP) for binary classification using Keras:
# Import necessary libraries
import numpy as np
import keras
from keras.models import Sequential
from keras.layers import Dense
# Generate dummy data for binary classification
x_train = np.random.random((1000, 20))
y_train = np.random.randint(2, size=(1000, 1))
x_test = np.random.random((100, 20))
y_test = np.random.randint(2, size=(100, 1))
# Create a sequential model
model = Sequential()
# Add a dense layer with 32 neurons and activation function "relu"
model.add(Dense(32, activation='relu', input_dim=20))
# Add a dense layer with 1 neuron and activation function "sigmoid"
model.add(Dense(1, activation='sigmoid'))
# Compile the model with binary crossentropy loss and the "adam" optimizer
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
# Train the model for 1000 epochs with a batch size of 32
model.fit(x_train, y_train, epochs=1000, batch_size=32)
# Evaluate the model on the test data
loss, accuracy = model.evaluate(x_test, y_test)
print("Test Loss: ", loss)
print("Test Accuracy: ", accuracy)
In this example, we start by generating dummy data for binary classification. The Sequential model is created and two dense layers are added. The first dense layer has 32 neurons and uses the rectified linear unit (ReLU) activation function, while the second dense layer has 1 neuron and uses the sigmoid activation function. The model is then compiled with binary crossentropy loss and the Adam optimizer. Finally, the model is trained for 1000 epochs with a batch size of 32 and evaluated on the test data. The test loss and accuracy are then printed to the console.
Keras is a powerful and user-friendly library for deep learning. Its simple API makes it easy to develop deep learning models, while its pre-trained models and support for fine-tuning allow developers to save time and resources compared to training models from scratch. Keras is a great tool for both experienced deep learning practitioners and those who are new to the field. For any assistance, contact us here.
Comments