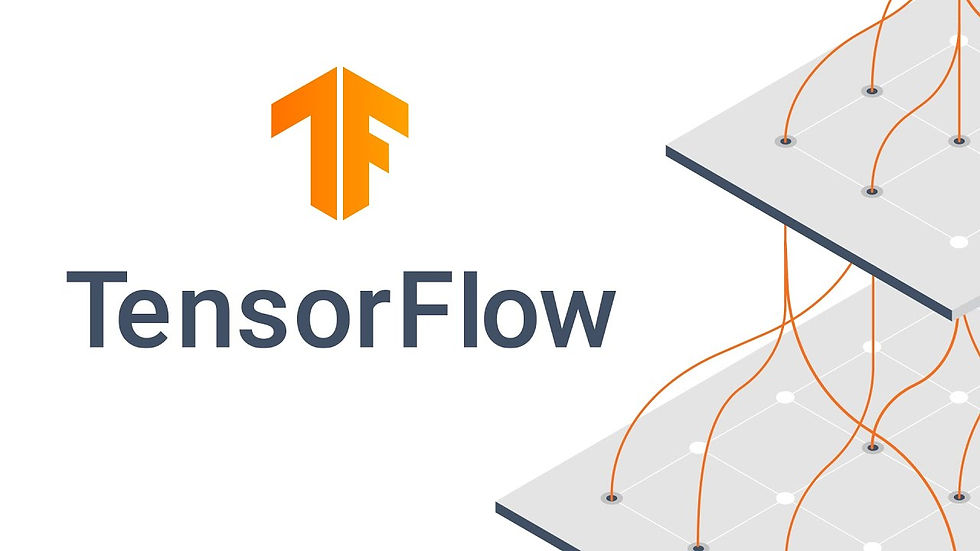
TensorFlow is a popular open-source software library for deep learning, developed by the Google Brain team. It is a comprehensive library that allows developers to build, train, and deploy artificial neural networks (ANNs) for a wide range of applications, from image recognition to natural language processing. TensorFlow is widely used for deep learning due to its flexibility, scalability, and ease of use.
In this article, we will explore the basics of TensorFlow and provide examples of code that demonstrate its capabilities.
What is TensorFlow?
TensorFlow is a software library that allows developers to build and train artificial neural networks. It provides a framework for building, training, and deploying complex models that can learn to perform tasks such as image recognition, text classification, and natural language processing.
TensorFlow is based on a graph-based computational model, where the data is processed by a series of operations (or nodes) in the graph. These nodes can be mathematical operations, activation functions, or even entire neural network layers. The data, represented as tensors, is passed through the graph, and the values of the tensors are updated after each operation. This process allows TensorFlow to perform backpropagation and update the parameters of the model during training.
How to use TensorFlow?
To use TensorFlow, you need to first install the library. TensorFlow is compatible with both Python and C++, and can be installed using pip or another package manager.
Once you have TensorFlow installed, you can start building and training your own deep learning models. The first step is to create a computational graph, which is a series of operations that define the structure of your model.
Next, you need to define the loss function, which is a metric that measures the performance of the model. This is typically a mean squared error or cross-entropy loss, depending on the task you are trying to perform.
Finally, you need to specify the optimizer, which is the algorithm that updates the parameters of the model during training. TensorFlow provides several optimizers, including the popular Stochastic Gradient Descent (SGD) and Adaptive Moment Estimation (Adam) algorithms.
Example of TensorFlow code
In this example, we will create a simple linear regression model to predict the output given an input. The code below shows how to define the computational graph and train the model.
import tensorflow as tf
import numpy as np
# Define the input data
x = np.array([1, 2, 3, 4])
y = np.array([0, -1, -2, -3])
# Define the model parameters
W = tf.Variable([0.3], dtype=tf.float32)
b = tf.Variable([-0.3], dtype=tf.float32)
# Define the input placeholder
x_ph = tf.placeholder(tf.float32)
# Define the model
linear_model = W * x_ph + b
# Define the loss function
loss = tf.reduce_sum(tf.square(linear_model - y))
# Define the optimizer
optimizer = tf.train.GradientDescentOptimizer(0
Comments